Updated on July 25, 2024
What is a IOS Chatbot?
An iOS chatbot is a computer program designed to simulate human conversation through text or voice interactions on devices running Apple’s iOS operating system. These chatbots are powered by artificial intelligence (AI) algorithms and are capable of understanding and responding to user queries and commands.
In this article, we will be sharing steps to building an iOS chatbot with Kompose chatbot builder. We will teach you everything you need to build a sample chatbot using Kompose for an iOS app.
Step by Step Guide to Building iOS Chatbot with Kommunicate
Step 1: Setup an account in Kommunicate
If you do not have an account in Kommunicate, you can create one for free.
Next, log in to your Kommunicate dashboard and navigate to the Bot Integration section. Locate the Kompose section and click on Integrate Bot.
If you want to build a bot from scratch, select a blank template and go to the Set up your bot section. Select the name of your bot, your bot’s Avatar, and your bot’s default language and click “Save and Proceed.”
You are now done creating your bot. Now comes the interesting part – training the bot for a query it does not understand. This is done by clicking the “Enable bot to human transfer” feature. Enable this feature and click “Finish Bot Setup.”
From the next page, you can choose if this bot will handle all the incoming conversations. Click on “Let this bot handle all the conversations,” and you are good to go.
Newly created bot here: Dashboard →Bot Integration → Manage Bots.
Step 2: Create welcome messages & answers for your chatbot
Go to the ‘Kompose – Bot Builder’ section and select the bot you created.
First, set the welcome message for your chatbot. The welcome message is the first message that the chatbot sends to the user who initiates a chat.
Click the “Welcome Message” section. In the “Enter Welcome message – Bot’s Message” box, provide the message your chatbot should be shown to the users when they open the chat, and then save the welcome intent.
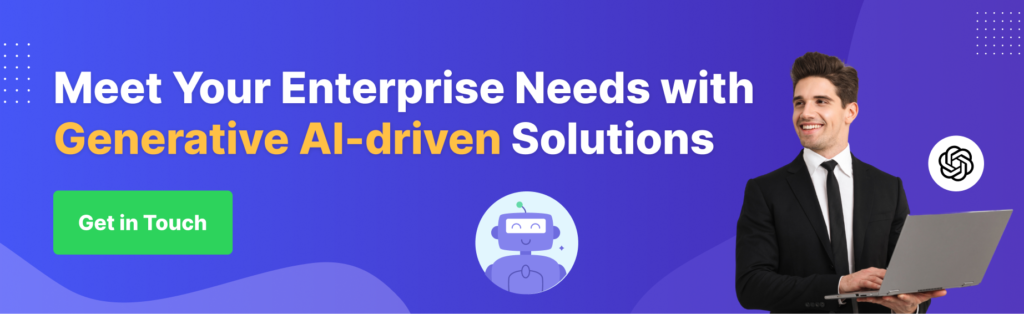
Once you have created the Welcome message, it is time to go to the “Settings” section of your Bot builder and toggle on ChatGPT.
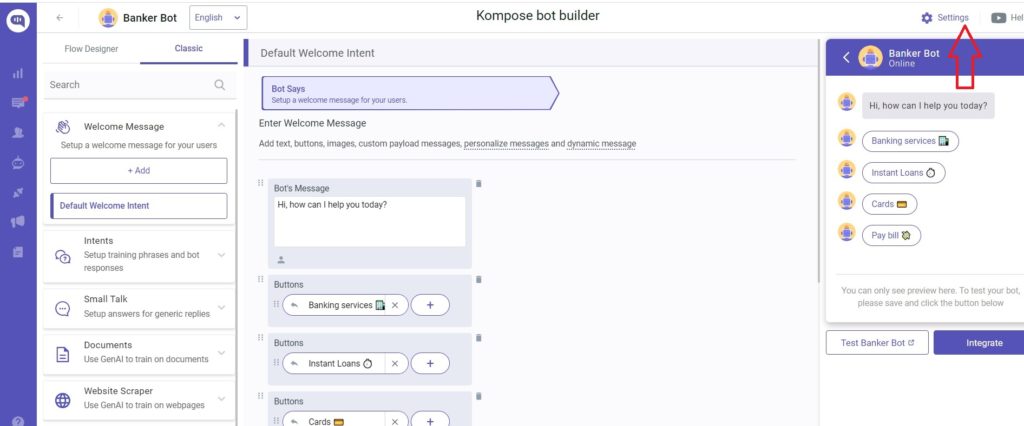
Now, just select “ChatGPT” from the panel and toggle on, and ChatGPT will now answer the questions that you pose to the chatbot.
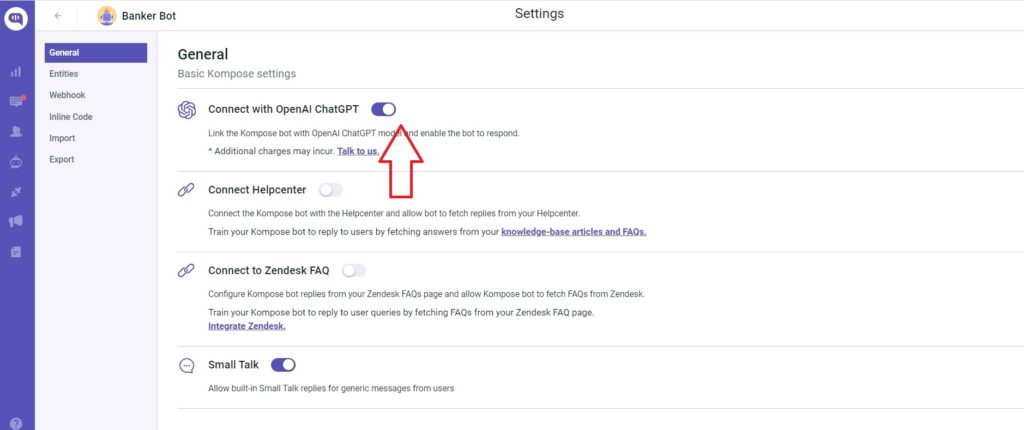
You have successfully integrated ChatGPT to your chatbot. It is now time to add this chatbot to your iOS project.
Phase 2: Add the created chatbot to your IOS Project using Cocoapods
Step 1: Setup Cocoapods:
Since we are going to add the Kommunicate SDK using cocoapods, this step is necessary for it. If you already have cocoapods in your system, then you can skip the step. If not, follow the link & install cocoapods.
Step 2: Create a iOS app :
You can create an app (my-app) from X code.
https://developer.apple.com/documentation/xcode/creating-an-xcode-project-for-an-app
Now project will look like this:
Step 3 : Create Podfile :
Now Close the Xcode and navigate to the my-app folder in the terminal & create a new podfile by using the below command.
touch Podfile |
After executing the command, a podfile will be added to your my-app folder.
Step 4: Install Kommunicate SDK to the app:
Now open that Podfile & add the below lines to the podfile
source ‘https://cdn.cocoapods.org/’ use_frameworks! platform :ios, ‘12.0‘ target ‘my-app my-app’ do pod ‘Kommunicate’ , ‘~> 6.6.0‘ end |
After adding those lines, save it. Go to the my-app folder in the terminal & install the Kommuncate pod by using this command
pod install |
Now you can see the Kommunicate SDK is being installed.
Congratulations!! You have successfully added Kommunicate SDK into your app.
Step 5: Add Kommunicate Code to the app:
Now open your project using the below command in the terminal.
open my-app.xcworkspace // replace project with your project name |
Your project structure will be like this & you can also see the Kommunicate pod under the pods section.
Now go to the Appdelegate.swift file, and import Kommunicate first.
import Kommunicate |
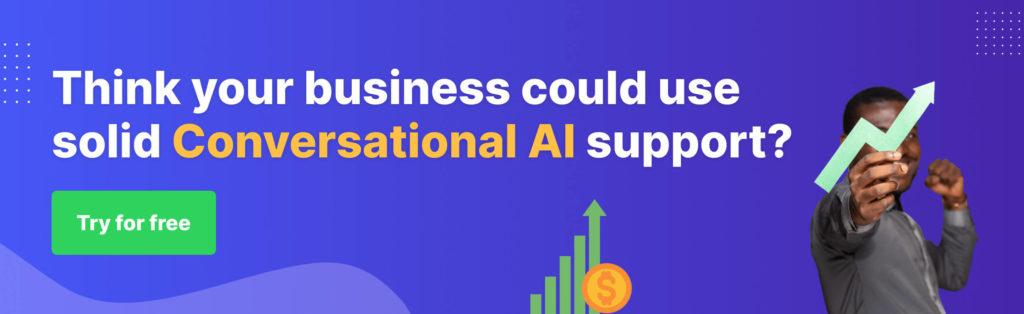
Add the below line inside the did Finish launching With Options method.
Kommunicate.setup(applicationId: “18ae6ce9d4f469f95c9c095fb5b0bda44”) // replace your appid |
You can get your app ID from Kommunicate Dashboard Install
Section(https://dashboard.kommunicate.io/settings/install)
Now your Appdelegate.swift file will look like this:
Next, we need to add a button which, when clicked, would open a conversation & a method to open Kommunicate when the user taps on the button.
Add these codes to create a button in your screen
let button = UIButton(frame: CGRect(x: 100,y: 100,width: 200,height: 60)) button.setTitle(“Launch Conversation”,for: .normal) button.setTitleColor(.systemBlue,for: .normal) button.addTarget(self,action: #selector(buttonAction),for: .touchUpInside) self.view.addSubview(button) |
Create a function for button action like this
@objc func buttonAction() { openConversation() } |
Next import Kommunicate using import Kommunicate and create a method for opening the conversation when tapped on the button.
func openConversation() { let userId = Kommunicate.randomId() let applicationId = “18ae6ce9d4f469f95c9c095fb5b0bda44” let kmUser = KMUser() kmUser.userId = userId kmUser.applicationId = applicationId Kommunicate.registerUser(kmUser, completion: { response, error in guard error == nil else { return } print(“Login Success”) Kommunicate.createAndShowConversation(from: self) { error in if error == nil { print(“Failed to launch the conversation”) } print(“Successfully launched the conversation”) } }) } |
That’s it. You have successfully completed the setup.Now Launch the app & click the button.
You can see the Kommunicate Chat Widget.
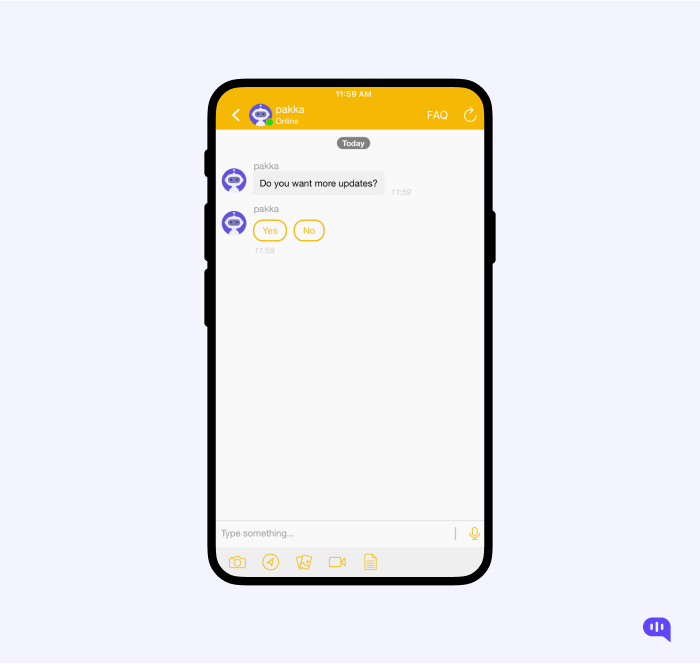
Finally, the ViewController Class looks like this:
import UIKit import Kommunicate class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let button = UIButton(frame: CGRect(x: 100,y: 100,width: 200,height: 60)) button.setTitle(“Launch Conversation”,for: .normal) button.setTitleColor(.systemBlue,for: .normal) button.addTarget(self,action: #selector(buttonAction),for: .touchUpInside) self.view.addSubview(button) } @objc func buttonAction() { openConversation() } func openConversation() { let userId = Kommunicate.randomId() let applicationId = “18ae6ce9d4f469f95c9c095fb5b0bda44” let kmUser = KMUser() kmUser.userId = userId kmUser.applicationId = applicationId Kommunicate.registerUser(kmUser, completion: { response, error in guard error == nil else { return } print(“Login Success”) Kommunicate.createAndShowConversation(from: self) { error in if error == nil { print(“Failed to launch the conversation”) } print(“Successfully launched the conversation”) } }) } } |
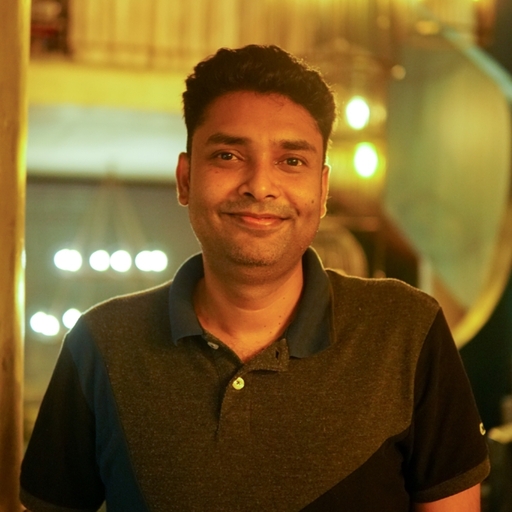
As a seasoned technologist, Adarsh brings over 14+ years of experience in software development, artificial intelligence, and machine learning to his role. His expertise in building scalable and robust tech solutions has been instrumental in the company’s growth and success.