Updated on February 12, 2025
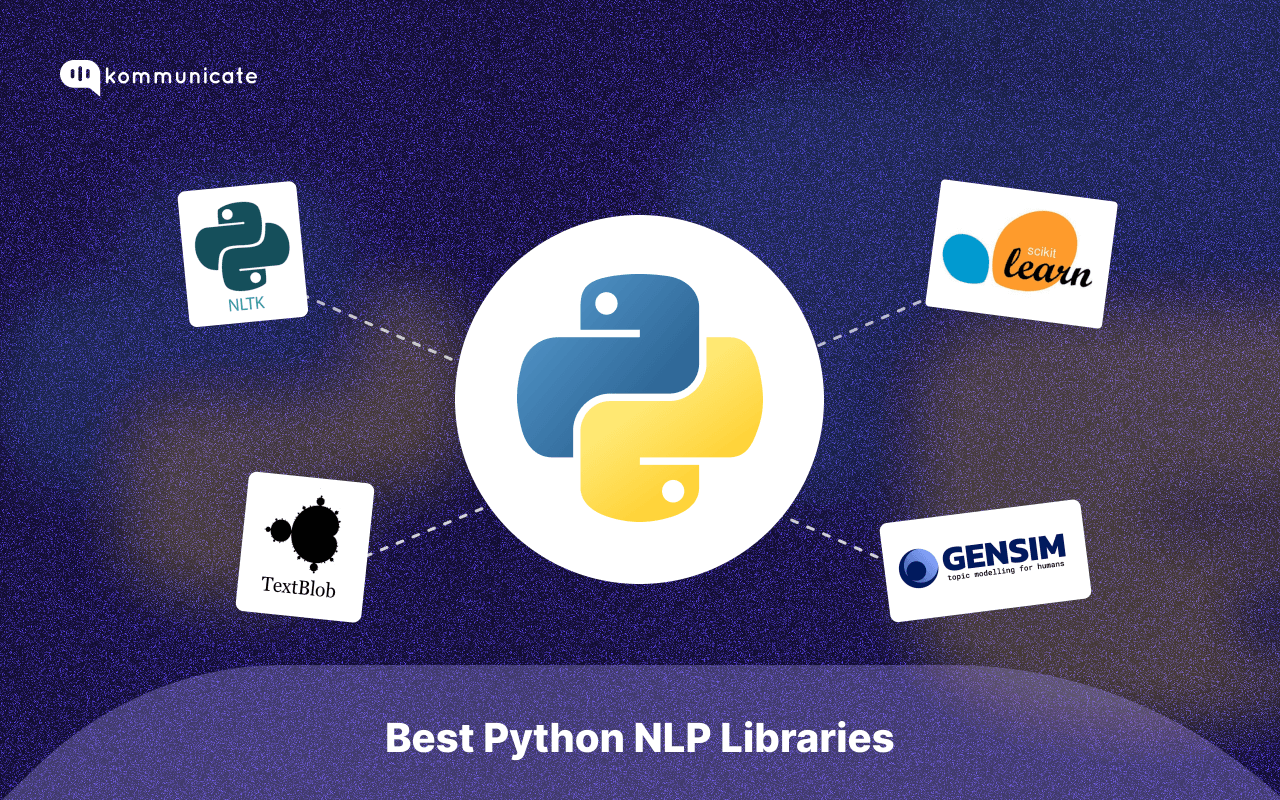
The recent rise in LLMs and various generative AI chatbots has also boosted the use of NLP across industries. While Natural Language Processing (NLP) has existed for decades, this is the first time the data and training around it has been so accessible.
Part of this accessibility is fueled by specialized NLP libraries that are available in Python. Several of these standardized programming libraries can run world-class NLP products at scale. Plus, most of these Python NLP libraries are free, allowing you to experiment with implementing NLP in your native applications in just a few steps.
In this blog, we’re exploring some of the best Python NLP libraries we have used.
1. NLKT
Realistically, NLKT is the first Python NLP library that you’ll use. Universities widely use it as a practical introduction to basic NLP concepts. It is a free, open-source library from the University of Pennsylvania and has a complimentary book that can be used to learn NLP concepts or teach students about them.
However, it’s hard to build production-ready apps using this library since it has many memory inefficiencies. It also has an easy-to-use interface that lets you navigate through 50 corpora and lexical resources.
Use-Cases of NLKT
You can use this library to run the following processes:
- Classification – You can classify texts natively with NLKT using Naive Bayes and Decision Tree algorithms.
- Tokenize – You can divide your texts into smaller parts (words).
- Stemming – You can generate the related words to a particular word. For example – “programmer” is related to “program.”
- Tagging – You can tag specific words as parts of speech using the library.
- Parsing – You can represent the syntactic structure of a particular text using trees.
- Semantic Reasoning – NLKT has a set of functions to perform semantic analysis and answer basic questions from a given text.
2. Gensim
Gensim is a memory-independent library for topic modeling. It’s widely used by developers worldwide and is a very efficient way to train vector embeddings.
It is so efficient because it uses NumPy’s BLAS (Basic Linear Algebra Subprograms) functions underneath the Python NLP libraries to enable matrix calculations at scale. It also uses data streaming algorithms that allow it to read only part of a data corpus at a time, helping it to process large amounts of data without exceeding RAM usage (hence the memory independence).
Use-Cases of Gensim
- Topic Modelling – The Gensim library specializes in topic modeling; it can identify words or phrases that occur together and sort them into different topics. This method is used to find the issues behind documents.
- Latent Semantic Indexing (LSA) – The library has several topic modeling ML algorithms built into it, and LSA is one of them. It can determine a document’s topic by calculating the word usage frequency across them and then use their co-occurrence to group documents.
- Latent Dirichlet Allocation (LDA) – Another topic modeling algorithm. LDA finds the topic of the input by associating words with particular issues and then sorting documents using this probability score.
- Word2vec and Doc2vec – The Python library is widely used in vector representations and has the famous word2vec and doc2vec algorithms.
- Calculate Similarity Matrices – The library can calculate the similarities between two inputs using cosine similarity algorithms.
- Summarization – Genshim can summarize a text by identifying the essential features of the document you have provided and then using them to create a smaller text.
3. TextBlob
TextBlob is also a free and open-source library in Python that can help you perform basic NLP operations. It has similar capabilities to NLKT and is very efficient for small-scale NLP projects.
TextBlob is computationally inexpensive and can be implemented quickly, making it an ideal choice for lightweight products or beginner NLP projects.
Use-Cases of TextBlob
- Part of Speech (POS) Tagging – Using NLP algorithms, TextBlob can automatically understand which parts of your inputs are nouns, verbs, adjectives, etc.
- Sentiment Analysis – TextBlob has functions that can understand a sentence’s sentiment and objectivity. For sentiment, it scores an input in the [-1,1], where a positive sentiment generates a positive score, and a negative sentiment generates a negative one.
For objectivity, it can analyze whether a sentence is an opinion, scoring in the range [0,1], with 1 representing a purely personal opinion. TextBlob does this analysis with built-in rules and is regarded as a heuristics-based system. - Classification – Like NLKT, this Python NLP library can classify texts using Naive Bayes and decision tree algorithms.
- Tokenization – The library allows you to parse an input into smaller parts (words) called tokens.
- Word and Phrase Frequencies – The library allows you to count the frequency of different words and phrases within your input text. This is important for further NLP algorithms that use these frequency numbers to understand the text.
- Parsing – TextBlob can build trees out of your input, parsing the order of the text provided.
- N-grams – TextBlob can break down your text into smaller n-grams (continuous sequences of texts, like two words that occur together). These n-grams are used to understand the semantics of the input text using advanced algorithms.
- Word Inflection – This library can pluralize (turn “word” into “words”) and singularize (“words” to “word”) and lemmatize them.
- Spelling Correction – TextBlob has functions that can identify and correct incorrectly spelled words.
4. Scikit-Learn
If TextBlob and NLKT are golden standards for teaching NLP to students, Scikit-Learn is the golden standard for a library that is used in products. The Google Summer of Code project debuted this library in 2007, and it has found widespread use in NLP applications everywhere.
The library uses NumPy, SciPy, and MatPlotLib to power its wide range of functions. It has a host of popular ML algorithms built into it and can be implemented into products because it can handle large-scale data.
Use-Cases of Scikit-Learn
- Classification – You can use Scikit-Learn to classify your input using popular ML algorithms, including Random Forest, nearest Neighbors, and Gradient Boosting.
- Regression – This Python library comes armed with several algorithms that can predict the future movement of some data. It can run everything from Naive Bayes and Decision tree algorithms to Stochastic Gradient Descent.
- Clustering – The library also has popular clustering algorithms that let you put similar values into chunks for easier data processing. Some popular algorithms that are available are k-means, HDC Scam, and hierarchical clustering.
- Dimensionality Reduction – Often, when you have large volumes of data, you need to reduce its dimensionality to maximize the signal from it. Scikit-Learn has several algorithms that allow you to reduce the dimensionality of the data you input. Some algorithms it uses are Principal Component Analysis (PCA) and Latent Semantic Analysis (LSA).
- Model Selection – The library offers flexibility in terms of predictive modeling. You can draw the estimates of the result a particular parameter influences and then learn the optimal hyperparameters for the model.
- Preprocessing – ML algorithms often assume that a normalized dataset is being presented to them and make errors when the data is not normalized. Preprocessing is the means by which you normalize a dataset that you feed into an ML algorithm. On Scikit-Learn, you can remove outliers from your dataset to ensure that an algorithm can run properly on your datasets.
5. Pattern
The Pattern Python library is well-known for its data mining and NLP capabilities. The library is robust and well-tested, making it suitable for many production-grade applications.
This presents you with an interesting opportunity. You can build applications that take texts from publicly-available websites and create applications using NLP. In Kommunicate, we do something similar for our FAQ Chatbots.
This Python NLP library comes armed with some standard NLP algorithms and is frequently updated with open-source contributions.
Use-Cases of Pattern
- Parsing – Like other libraries in this list, Pattern also has algorithms to divide your input and structure it using trees.
- N-grams – Just like TextBlob, Pattern also has algorithms for dividing your input data into smaller n-grams for NLP.
- Sentiment Analysis – The pattern library includes several popular algorithms like LSA and LDA to understand the sentiment of different inputs.
- Spelling Check – you can use Pattern’s functions to identify and correct misspelled words in the input.
- Frequency Checks – You can use Pattern to estimate the frequency of occurrence of a particular word or phrase in the input.
- Changing the Degree of an Adjective – You can use Pattern’s functions to change an adjective from your input into the superlative and comparative degrees.
- Pluralization & Singularization – Just like TextBlob and NLKT, you can pluralize and singularize words using Pattern’s library.
Enhance support workflows, accelerate resolutions, and boost efficiency with AI-powered email ticketing from Kommunicate
Also Read: 6 Best NLP Libraries for Node.js and JavaScript
Also Read: How to Extract Name Using NLP
Also Read: How to Create a Healthcare Chatbot Using NLP
Some Notes on NLP Libraries
As the use cases for NLPs expand and new algorithms come out, understanding the basic tooling behind these processes will be crucial for any programmer. And if you’re someone building a production-grade NLP applications (like Kommunicate), you’ll need a good handle on Python.
The libraries listed here let you do just that. Python NLP Libraries like NLKT, TextBlob, and Pattern provide learners with hands-on experience building production-grade AI applications.
On the other hand, Gensim and Scikit-Learn have become central to many AI-based applications across the world.
Remember to follow documentation and use the tutorials available with each Python NLP library to launch new projects. Iterating and becoming better is how we will unlock the next level of NLP and AI applications.
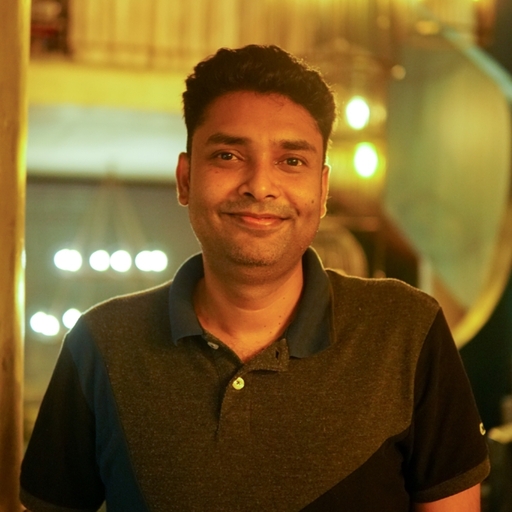
As a seasoned technologist, Adarsh brings over 14+ years of experience in software development, artificial intelligence, and machine learning to his role. His expertise in building scalable and robust tech solutions has been instrumental in the company’s growth and success.